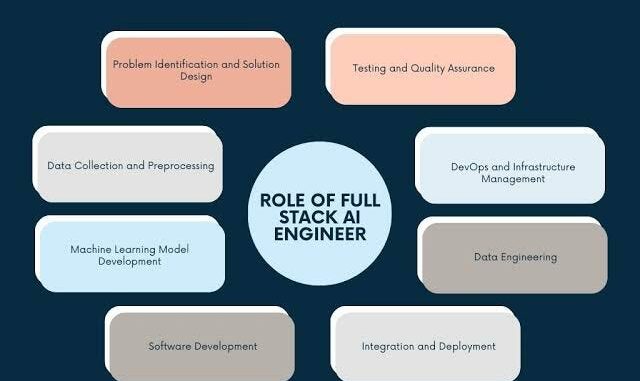
In modern web development, AI and ML are transforming how we build and maintain applications. Let’s walk through a practical example of developing an e-commerce platform and explore how AI/ML can be integrated into various aspects of the application with code snippets and samples.
Scenario: Building an E-Commerce Platform
You’re building a new e-commerce platform where users can browse products, make purchases, and interact with customer support. Here’s how AI/ML can enhance different features of this platform.
1. Intelligent Code Assistance
How It Works: AI-powered code assistants like GitHub Copilot suggest code snippets and functions based on your project context.
Example Code:While integrating a payment gateway, you might need to handle secure transactions. GitHub Copilot can suggest code for payment integration
```javascript
// Example of using a payment gateway API
const paymentGateway = require(’payment-gateway-sdk’);async function processPayment(amount, paymentDetails) {
try {
const response = await paymentGateway.process({
amount: amount,
cardNumber: paymentDetails.cardNumber,
expiryDate bi: paymentDetails.expiryDate,
cvv: paymentDetails.cvv,
});
return response;
} catch (error) {
console.error(’Payment processing failed’, error);
throw error;
}
}
```
2. Enhanced User Experience with Personalization
How It Works:ML algorithms analyze user behavior to deliver personalized recommendations.
Example Code:Using TensorFlow.js for product recommendations based on user browsing history.
```javascript
// TensorFlow.js for personalized product recommendations
import * as tf from '@tensorflow/tfjs';async function recommendProducts(userHistory) {
// Load pre-trained model
const model = await tf.loadLayersModel('model/model.json');
// Prepare user data
const userData = tf.tensor([userHistory]);
// Make predictions
const recommendations = model.predict(userData);
// Process and return recommended products
return recommendations.arraySync();
}
```
3. Automated Testing and Debugging
How It Works:AI tools automate testing and debugging by adapting to changes in the application.
Example Code:Using Testim.io or similar tools to run automated tests.
```javascript
// Example of a test script using Testim.io
describe('Order Tracking Feature', () => {
it('should display order status correctly', () => {
cy.visit('/order-tracking');
cy.get('input[name="orderId"]').type('12345');
cy.get('button[type="submit"]').click();
cy.get('.order-status').should('contain', 'Shipped');
});
});
```
4. Smarter Analytics and Insights
How It Works:AI analytics tools process data to provide actionable insights.
Example Code: Using Google Analytics API to track user engagement.
```javascript
// Google Analytics API example
const analytics = require('google-analytics-api');
async function trackUserEngagement(userId, event) {
await analytics.trackEvent({
userId: userId,
eventCategory: 'User Engagement',
eventAction: event.action,
eventLabel: event.label,
});
}
```
5. Natural Language Processing (NLP) for Enhanced Interactions
How It Works:NLP technologies enhance user interactions through chatbots or virtual assistants.
Example Code: Using the `dialogflow` library to create a chatbot for customer support.
```javascript
// Example of integrating Dialogflow for customer support
const dialogflow = require(’@google-cloud/dialogflow’);
const sessionClient = new dialogflow.SessionsClient();
const projectId = 'your-project-id’;async function detectIntent(sessionId, query) {
const sessionPath = sessionClient.projectAgentSessionPath(projectId, sessionId);const request = {
session: sessionPath,
queryInput: {
text: {
text: query,
languageCode: 'en-US’,
},
},
};const responses = await sessionClient.detectIntent(request);
const result = responses[0].queryResult;
return result.fulfillmentText;
}
```
Integrating AI and ML into your full-stack development projects can significantly enhance the functionality and user experience of your applications. By leveraging intelligent code assistants, personalized recommendations, automated testing, smarter analytics, and NLP, you can streamline development, improve application performance, and deliver a more engaging experience for users. The code samples provided demonstrate practical implementations of these technologies, showcasing their potential to transform your projects.
Be the first to comment