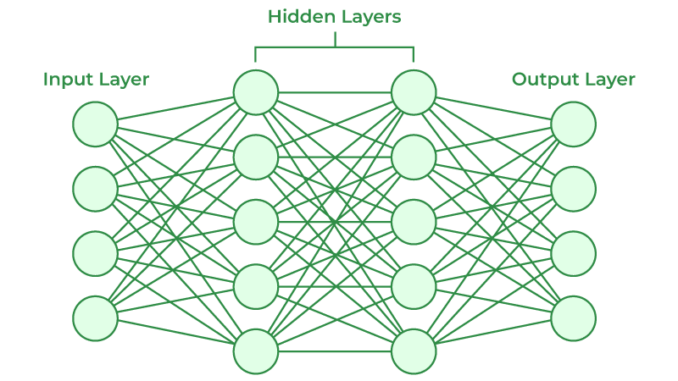
In the rapidly evolving field of artificial intelligence, deep learning stands out as one of the most transformative technologies. By mimicking the human brain, deep learning models can recognize patterns in vast amounts of data, enabling groundbreaking advancements in areas like image recognition, natural language processing, and beyond. In this article, we’ll explore the basics of deep learning, get you set up with TensorFlow and Keras, and guide you through building and training your first neural network.
Deep learning is a subset of machine learning that utilizes neural networks with many layers (hence the term “deep”). These layers enable the model to learn from data in a hierarchical fashion, extracting increasingly complex features at each layer. This approach is particularly powerful for tasks involving large datasets and complex patterns.
A neural network is composed of layers of interconnected nodes, or neurons. Each neuron takes an input, applies a weighted sum and an activation function, and passes the output to the next layer. The network learns by adjusting these weights based on the error in its predictions, a process called backpropagation.
Here’s a simple diagram of a neural network:
- Layers: Collections of neurons. Common types include dense (fully connected) layers, convolutional layers (for image data), and recurrent layers (for sequential data).
- Activation Functions: Functions that introduce non-linearity into the model, enabling it to learn complex patterns. Examples include ReLU, sigmoid, and tanh.
- Loss Function: Measures the error between the model’s prediction and the actual target. Common loss functions include mean squared error (MSE) for regression and categorical cross-entropy for classification.
- Optimizer: Algorithm that adjusts the model’s weights to minimize the loss function. Popular optimizers include SGD, Adam, and RMSprop.
TensorFlow and Keras are two popular frameworks for building and training deep learning models. TensorFlow is an open-source platform developed by Google, while Keras is a high-level neural networks API, written in Python and capable of running on top of TensorFlow.
To get started, you’ll need to install TensorFlow and Keras. You can do this easily using pip:
pip install tensorflow keras
After installation, verify that TensorFlow and Keras are correctly installed by running the following Python code:
import tensorflow as tf
from tensorflow import kerasprint(tf.__version__)
print(keras.__version__)
If you see version numbers for both TensorFlow and Keras, you’re good to go!
Let’s build a simple neural network to classify handwritten digits from the famous MNIST dataset. This dataset contains 60,000 training images and 10,000 test images of handwritten digits (0–9), each represented as a 28×28 pixel grid.
import tensorflow as tf
from tensorflow.keras import layers, models
import matplotlib.pyplot as plt# Load the MNIST dataset
(train_images, train_labels), (test_images, test_labels) = tf.keras.datasets.mnist.load_data()
# Normalize the images to [0, 1] range
train_images = train_images / 255.0
test_images = test_images / 255.0
We’ll create a simple sequential model with two dense (fully connected) layers. The first layer will have 128 neurons with a ReLU activation function, and the second layer will be the output layer with 10 neurons (one for each digit) and a softmax activation function.
model = models.Sequential([
layers.Flatten(input_shape=(28, 28)), # Flatten the 28x28 images into 1D vectors
layers.Dense(128, activation='relu'),
layers.Dense(10, activation='softmax')
])
Next, we need to compile the model by specifying the optimizer, loss function, and metrics to monitor.
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
We can now train the model using the training data. We’ll also validate the model on the test data to see how well it generalizes.
model.fit(train_images, train_labels, epochs=5, validation_data=(test_images, test_labels))
Finally, let’s evaluate the model’s performance on the test dataset.
test_loss, test_acc = model.evaluate(test_images, test_labels)
print(f'Test accuracy: {test_acc}')
Congratulations! You’ve just built and trained your first neural network using TensorFlow and Keras. This is just the beginning — deep learning has a vast array of techniques and architectures to explore. With TensorFlow and Keras, you have powerful tools at your disposal to dive deeper into the world of artificial intelligence.
Feel free to share your experiences and insights in the comments below. Happy coding!
Be the first to comment