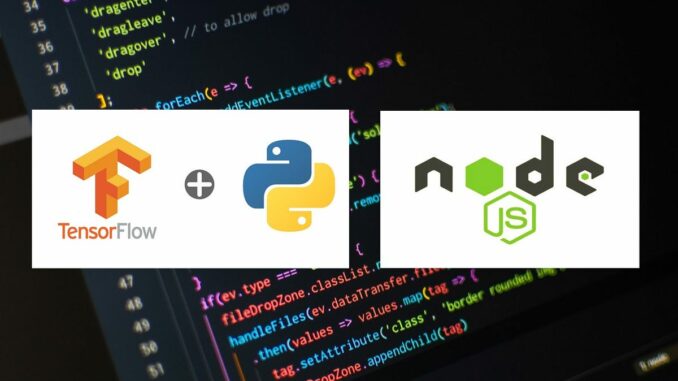
Hey there, curious coder! it’s me again, the rocket lover 🚀
Ever scrolled through a playlist and thought, “How did they know I’d like this song?” or been impressed when your email sorted out that ad-email as spam?
That’s Machine Learning (ML) right there. It’s making our apps smarter, our decisions faster, and in general our lives a bit cooler.
I have implemented Machine Learning in a few of my Node.js apps, so I figured it would be good to write an article on how I go about it.
Node.js, with its asynchronous capabilities, isn’t just about creating web servers. It’s about scalability, speed, and yes, serving some cool super-smart ML models. Pairing ML with Node.js is like giving your app a brain boost. It’s about making it not just function but also think and adapt.
Think of Machine Learning as teaching your computer a new trick, only the trick can be as simple as recognizing a cat or as complex as predicting in what year will humans land on Mars.
How Do ML Models Work?
Imagine you’re training your app to distinguish between two types of rockets, let’s say, the Falcon-9 and the Saturn-V. You show your app a lot of images of both rockets, from launch sequences to engine designs. Over time, the app begins to recognize the unique signs that distinguish one from the other. This moment, where your app can confidently differentiate between the two without your help, is basically your ML model in action. We call this phase ‘training’.
Different Flavors (Types) of Models
- Classification: This is like the Falcon-9 vs. Saturn-V game. It’s about categorizing things. In a broader scope, it could be about identifying the genre of a song or figuring out whether an email is spam or not.
- Regression: Now, we’re talking numbers. Like, based on fuel type and payload, how far will this rocket travel? Or, given a spaceship’s age and design, what’s its maximum speed?
- Clustering: Grouping stuff based on similarities. Imagine sorting a box of assorted candies by color or taste.
- Reinforcement Learning: Training a rover to navigate Mars. Each correct move earns a reward (like discovering water!), and every tumble in a sand hole? A lesson learned.
Training, Testing, and Validation — The Learning Curve
Typically, you can’t just throw data at a model and hope for the best. It’s a process:
- Training: Show the model lots of data and let it learn. Think of this as the study phase before an exam.
- Validation: Check how it’s doing with some new data, tweak if necessary. It’s the quick quiz before the final test.
- Testing: Time to put it to the real test. Unseen data comes in, and you measure how well the model’s predictions are.
Once your model succeeds all these stages, you’ve got a system that doesn’t just operate on pre-fed instructions but makes intelligent decisions based on patterns it’s learned (you’ve taught it).
If you are thinking about Python, I absolutely understand as it often steals the spotlight when we talk about ML, but Node.js has its secret weapons too. Here’s how I’ve integrated ML into my Node.js apps.
TensorFlow.js
While TensorFlow.js started as a way to run pre-trained models in the browser, there’s a Node.js package that lets us use it server-side. This opens the door to training and running models in a Node.js environment, utilizing the server’s computational power.
Let’s build a rocket classifier. We’ll train a simple model to predict if a given rocket is either a Falcon-9 or a Saturn-V based on a few features.
Setup: First things first, we need to install the Node.js variant of TensorFlow.js
npm install @tensorflow/tfjs-node
Loading and Preprocessing Data:
For the sake of simplicity, let’s assume we have data in a JavaScript array. In a real-world scenario, we’d likely fetch this from a database or a file.
const tf = require('@tensorflow/tfjs-node');const data = [
{ features: [70, 1, 15], label: 'Falcon-9' },
// 70 meters tall, fuelType=1 (let's say liquid oxygen), 15 tons payload
{ features: [110, 1, 140], label: 'Saturn-V' },
{ features: [72, 1, 18], label: 'Falcon-9' },
{ features: [112, 0, 130], label: 'Saturn-V' },
//... more data
];
// Convert data to tensors
const xs = tf.tensor2d(data.map(item => item.features));
const ys = tf.tensor2d(data.map(item => (item.label === 'Falcon-9' ? [1, 0] : [0, 1])));
But often, I’ve found it useful to train my models using robust setups (like using TensorFlow in Python) and then bring them into my Node.js apps. If you’ve got a pre-trained model you can load it like this:
const tf = require('@tensorflow/tfjs-node');const model = await tf.loadLayersModel('file://path/to/my-model.json');
Building the Model: Setting up a simple neural network to distinguish between the rockets.
const model = tf.sequential();model.add(tf.layers.dense({units: 128, activation: 'relu', inputShape: [ 3 /* number of features you have */]})); // We have 3 features
model.add(tf.layers.dense({units: 2, activation: 'softmax'}));
model.compile({loss: 'categoricalCrossentropy', optimizer: tf.train.adam(), metrics: ['accuracy']});
Training the Model: Now we train the model using our data.
async function train() {
await model.fit(xs, ys, {epochs: 50}); // Increased epochs for a small dataset
console.log('Rocket model trained!');
}
train();
Always ensure that the async/await or promises are correctly handled. This keeps things smooth and prevents surprises 🤭
Making Predictions: After training, we can predict the class of new rockets. For example a new rocket with the features height: 75 meters
, fuelType: 1
, and payload: 20 tons
.
const newRocketFeatures = tf.tensor2d([[75, 1, 20]]);
const prediction = model.predict(newRocketFeatures);
console.log(prediction.dataSync()); // Outputs likelihoods for Falcon-9 and Saturn-V
Real-Time Training: Sometimes, we might want our app to learn on-the-go, adjusting to new data. Suppose users of our space-themed app can submit their own rocket specifications and correct the app’s predictions, providing valuable new data. Every time we accumulate a significant batch of new data, we can retrain the model.
async function realTimeTrain(newData) {
const newXS = tf.tensor2d(newData.map(item => item.features));
const newYS = tf.tensor2d(newData.map(item => (item.label === 'Falcon-9' ? [1, 0] : [0, 1])));await model.fit(newXS, newYS, {epochs: 50});
console.log('Model updated with new data!');
}
Dealing with Large Datasets: When dealing with large datasets, feeding all the data into the model at once might cause memory issues. TensorFlow.js provides functionality to handle data in smaller batches.
const batchSize = 32;
const numBatches = Math.ceil(data.length / batchSize);for (let i = 0; i < numBatches; i++) {
const batch = data.slice(i * batchSize, (i + 1) * batchSize);
const batchXS = tf.tensor2d(batch.map(item => item.features));
const batchYS = tf.tensor2d(batch.map(item => (item.label === 'Falcon-9' ? [1, 0] : [0, 1])));
await model.trainOnBatch(batchXS, batchYS);
console.log(`Completed batch ${i + 1}/${numBatches}`);
}
Saving and Reloading Models: After training or updates, we’d want to save the model to avoid redoing the work. TensorFlow.js provides convenient methods for this.
// Saving the model
const saveResult = await model.save('file://path/to/save/location');// Loading the saved model
const loadedModel = await tf.loadLayersModel('file://path/to/saved/model.json');
Note: By the way, when I first built a recommendation system for a school management app (UjuziApp). Initially, I considered using Python for the ML part. However, using TensorFlow.js with Node.js made the entire process seamless. No need to change between languages or set up communication channels between different backends, TensorFlow.js gets the job done just fine.
Python & Node.js
Sometimes we might want to train our model using Python’s extensive ML libraries, here is a hybrid approach I use.
Child Processes: This is a quick method I sometimes use for lightweight tasks.
const { spawn } = require('child_process');
const pythonProcess = spawn('python', ['path_to_python_script.py', arg1, arg2]);pythonProcess.stdout.on('data', (data) => {
// Handle your ML model's output here
console.log(`Model output: ${data}`);
});
Web API Approach: For heavier tasks and when scalability matters, I go for the API route. Using Flask in Python to serve the model.
from flask import Flask, request, jsonify
from keras.models import load_modelapp = Flask(__name__)
model = load_model('my_model.h5')
@app.route('/predict', methods=['POST'])
def predict():
data = request.json
prediction = model.predict([data])
return jsonify(prediction.tolist())
if __name__ == '__main__':
app.run(port=5000)
Then, in my Node.js app, I make a request to this API
async function getPrediction(data) {
const response = await axios.post('http://localhost:5000/predict', data);
return response.data;
}
Note: There was this one time I was building a recommendation system for an Insurance managerial app (MSE). I started with the child process approach. It worked like a charm initially. But as the member base grew, I had to switch to the web API method. It allowed me to separately scale the ML component from the main app, ensuring smoother performance.
Using ML with Node.js has been quite an adventure for me. There’s always something new to learn and experiment with. Dive in, play around, and don’t be afraid to mix and match tools to find what works best for your application.
Throughout our exploration, we dived deep into the power of TensorFlow.js within Node.js, but we shouldn’t forget the incredible combination of Python & Node.js. Many times, especially when working with sophisticated models developed in Python, creating a bridge between the two ecosystems can lead to a seamless and powerful integration, ensuring that you get the best of both worlds.
Let me share a tangible personal experience that shows the transformative potential of this technology. I collaborated with a school that relied on a digital platform to streamline interactions among teachers, students, and parents (UjuziApp). Recognizing the opportunity within the platform’s vast data, we introduced Machine Learning to select personalized learning paths. The initial model directed students towards resources that complemented their unique strengths and weaknesses.
However, it became much more interesting when we incorporated real-time model training. The system learned from the continuous feedback of teachers, refining its recommendations each week.
This journey, from ideation to tangible impact, represents the promise that Machine Learning, whether through Node.js directly or in combination with Python, brings to the table. As developers, it’s an exciting era where our creations aren’t just static entities; they evolve, learn, and adapt to better serve their purpose.
If this article has piqued your interest, I encourage you to dive deeper, the world of Machine Learning is vast and evolving, so integrate it into your Node.js projects, experiment with models, play with data, and most importantly, stay curious.
Until next time, keep coding, keep learning! and I will keep loving rockets 🚀
Be the first to comment