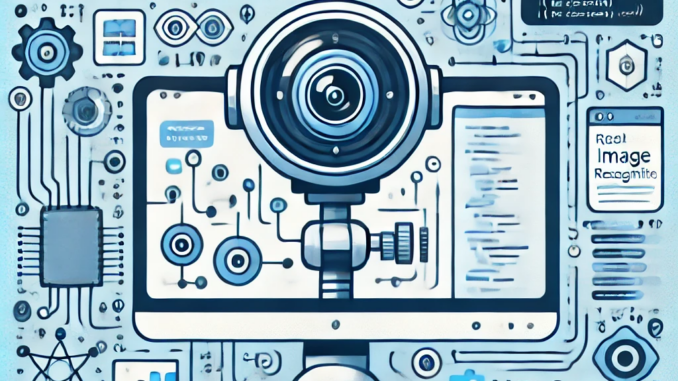
In the rapidly evolving field of artificial intelligence, real-time image recognition stands out as a significant innovation. Integrating .NET Core with TensorFlow, a leading open-source platform for machine learning, can provide powerful capabilities for building real-time image recognition applications. This article explores advanced techniques and real-world examples to illustrate how to achieve this integration effectively.
Setting Up the Environment
Step 1: Create a New .NET Core Project
First, create a new .NET Core console application:
dotnet new console -n RealTimeImageRecognition
cd RealTimeImageRecognition
Step 2: Add Required NuGet Packages
Add the TensorFlow.NET and SciSharp.TensorFlow.Redist packages to your project:
dotnet add package TensorFlow.NET
dotnet add package SciSharp.TensorFlow.Redist
Step 3: Install TensorFlow
Ensure you have TensorFlow installed. You can download and install it from the TensorFlow.
Implementing Real-Time Image Recognition
Step 4: Load and Preprocess the Model
Load a pre-trained TensorFlow model for image recognition. For this example, we’ll use a MobileNet model, which is optimized for mobile and real-time applications.
using System;
using Tensorflow;
using static Tensorflow.Binding;
using Tensorflow.NumPy;public class ImageRecognition
{
private readonly string modelFile = "mobilenet_v2_1.4_224_frozen.pb";
private readonly string labelsFile = "labels.txt";
private readonly int inputHeight = 224;
private readonly int inputWidth = 224;
private readonly string inputMean = "128";
private readonly string inputStd = "128";
private Graph graph;
private Session session;
public ImageRecognition()
{
graph = new Graph().as_default();
graph.Import(modelFile);
session = tf.Session(graph);
}
}
Step 5: Load and Preprocess Images
Implement a method to load and preprocess images before feeding them into the model:
public TFTensor LoadImage(string filePath)
{
var bitmap = new Bitmap(filePath);
var resized = new Bitmap(bitmap, new Size(inputWidth, inputHeight));
var matrix = new NDArray(new Shape(1, inputHeight, inputWidth, 3));for (int y = 0; y {
for (int x = 0; x {
var color = resized.GetPixel(x, y);
matrix[0, y, x, 0] = (color.R - float.Parse(inputMean)) / float.Parse(inputStd);
matrix[0, y, x, 1] = (color.G - float.Parse(inputMean)) / float.Parse(inputStd);
matrix[0, y, x, 2] = (color.B - float.Parse(inputMean)) / float.Parse(inputStd);
}
}
return matrix;
}
Step 6: Perform Inference
Run the preprocessed image through the model to get predictions:
public string[] ReadLabels()
{
return File.ReadAllLines(labelsFile);
}public string Predict(string imagePath)
{
var tensor = LoadImage(imagePath);
var results = session.run(graph.OperationByName("MobilenetV2/Predictions/Reshape_1"), new FeedItem(graph.OperationByName("input"), tensor));
var probabilities = results.First().ToArray();
var labels = ReadLabels();
var bestIndex = Array.IndexOf(probabilities, probabilities.Max());
return labels[bestIndex];
}
Step 7: Integrate with Real-Time Data Source
For real-time image recognition, integrate the image loading and prediction logic with a real-time data source, such as a webcam feed. Use an appropriate library for capturing images from the webcam, like OpenCV or AForge.NET.
Example: Using OpenCV to Capture Webcam Images
Install OpenCV:
pip install opencv-python
Capture images from the webcam and pass them to the TensorFlow model for prediction:
using OpenCvSharp;public void RecognizeFromWebcam()
{
using var capture = new VideoCapture(0);
using var window = new Window("Real-Time Image Recognition");
var image = new Mat();
while (true)
{
capture.Read(image);
if (image.Empty())
break;
var filePath = "frame.jpg";
image.SaveImage(filePath);
var label = Predict(filePath);
Cv2.PutText(image, $"Prediction: {label}", new Point(10, 30), HersheyFonts.HersheySimplex, 1.0, Scalar.White, 2);
window.ShowImage(image);
if (Cv2.WaitKey(1) == 27)
break; // Exit on 'ESC' key press
}
}
Integrating TensorFlow with .NET Core for real-time image recognition provides a powerful toolset for developing advanced AI applications. By leveraging pre-trained models, you can quickly implement image recognition capabilities and enhance them with real-time data sources such as webcams. This approach not only demonstrates the versatility of .NET Core but also highlights the potential of combining it with TensorFlow for cutting-edge AI solutions.
Be the first to comment