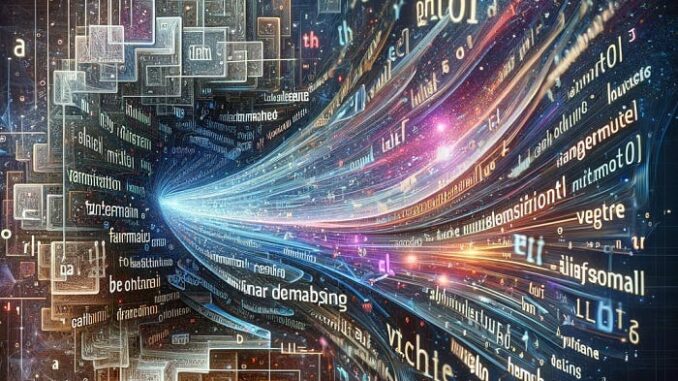
In the world of Artificial Intelligence (AI) and Language Learning Models (LLM), the term “vector embeddings” has become increasingly prominent. This concept is fundamental to understanding how machines process and comprehend human language. But what exactly does it mean and how is it applied, especially in the context of programming in JavaScript?
Vector embeddings are a natural language processing technique that involves converting words or phrases into numerical vectors in a fixed-dimensional space. This transformation allows computers to understand and process natural language in a more efficient and meaningful way.
Imagine each word in a language represented as a point in a multi-dimensional space. Words with similar meanings or contexts are closer to each other in this space. For example, “cat” and “dog” might be closer than “cat” and “airplane”.
In AI, especially in LLMs like GPT (Generative Pretrained Transformer), vector embeddings are crucial. They enable the model to capture the semantics of words and use this information to generate coherent and contextually relevant responses. These models are trained with huge amounts of text, learning the relationships between words and their contexts.
JavaScript, being one of the most popular programming languages, has also joined the AI trend. There are libraries like TensorFlow.js that allow the implementation of machine learning models in the browser or on the server with Node.js.
Here are some useful resources and libraries for getting started with vector embeddings in JavaScript:
- TensorFlow.js: A library for training and deploying ML models directly in the browser. More information here.
- ML5.js: Based on TensorFlow.js, this library offers a more friendly API for artists and beginners. Explore ML5.js.
- Natural: A Node.js module for natural language processing that includes support for vector embeddings. Visit Natural on NPM.
Step 1: Environment Setup
First, you need to have Node.js installed on your system. Then, create a new Node.js project and add the natural
library:
mkdir vector-embeddings-example
cd vector-embeddings-example
npm init -y
npm install natural
Step 2: Script Creation
Create a file called index.js
and open it in your favorite code editor.
Step 3: Code for Vector Embeddings
Next, write the following code in index.js
. This example shows how to use vector embeddings to find words similar in terms of their semantics:
const natural = require('natural');
const wordnet = new natural.WordNet();
// Function to find synonyms using WordNet
function findSynonyms(word) {
wordnet.lookup(word, function(results) {
results.forEach(function(result) {
console.log('Synonyms for', word, ':', result.synonyms);
});
});
}
// Example usage
findSynonyms('happy');
This code uses WordNet through the natural
library to search for synonyms of the word “happy”. WordNet is a lexical database that relates words according to their meaning, allowing us to explore vector embeddings at a basic level.
Step 4: Run the Script
Finally, run the script in your terminal:
node index.js
You will see a list of synonyms for the word “happy”. This is a basic example and the beginning of what you can do with vector embeddings and NLP in JavaScript.
This is a very simple and basic example to illustrate the concept. In more advanced applications, you might use TensorFlow.js or ML5.js to work with more complex machine learning models and perform vector embeddings on a large scale.
Vector embeddings can be applied in a variety of business contexts to improve decision-making, operational efficiency, and customer experience. Here are some examples of how they can be used in different business areas:
- Product Recommendation In e-commerce and streaming platforms, vector embeddings can be used to recommend products or content. By analyzing users’ purchase history or viewing habits and comparing it with other products or content, personalized recommendations can be generated that increase customer satisfaction and sales.
- Sentiment Analysis in Customer Reviews Companies can use vector embeddings to analyze the sentiment behind customer reviews and comments. This helps identify trends and recurring issues, improving the quality of the product or service and the customer experience.
- Customer Support Classification Vector embeddings can help to automatically classify customer support inquiries and direct them to the correct department. This can improve the efficiency of customer service and reduce response times.
- Market Trend Analysis Companies can use vector embeddings to analyze large amounts of market data, identifying trends and patterns that would not be obvious at first glance. This is useful for strategic decision-making, such as identifying market opportunities or predicting changes in demand.
- Improving Internal Search Systems Vector embeddings can improve the relevance and accuracy of internal search systems on websites and applications, helping users to quickly find the product or information they are looking for.
- Social Media Analysis Companies can use vector embeddings to analyze social media data, identifying key influencers, opinion trends, and the effectiveness of marketing campaigns.
- Fraud Detection In the financial sector, vector embeddings can be used to detect patterns of fraudulent transactions, improving security systems and reducing losses due to fraud.
- Marketing Personalization Vector embeddings can help personalize marketing campaigns, segmenting customers into groups with similar interests and behaviors and adjusting marketing messages to resonate better with each segment.
Currently, OpenAI does not provide a specific API to work directly with vector embeddings in its language models, such as GPT-3 or GPT-4. However, I can give you a conceptual example of how vector embeddings might be used in the context of an OpenAI language model.
Objective: Classify customer comments into categories such as “positive,” “negative,” or “neutral” using GPT-3 or GPT-4.
Process:
- Data Preparation: Collect a set of customer comments.
- Interaction with the Language Model:
- Input: A customer comment.
- Output: A text generated by the model indicating the sentiment category (positive, negative, neutral).
3. Implementation:
- Use the OpenAI API to send the comment to the GPT-3 or GPT-4 model.
- Configure the prompt so that the model understands it needs to classify the sentiment of the comment.
- Receive the response from the model and use it to determine the sentiment category.
Python Code Example:
openai.api_key = 'your_api_key'
response = openai.Completion.create(
engine="text-davinci-003",
prompt="Classify the following comment as positive, negative, or neutral: 'The customer service was exceptional, and the product exceeded my expectations.'",
max_tokens=60
)
category = response.choices[0].text.strip()
print("Sentiment Category:", category)
Note: This is a simplified example. In practice, it would be necessary to adjust the prompt and possibly train a specific model to achieve accurate and consistent results.
In this example, although we are not interacting directly with vector embeddings, the OpenAI language model uses them internally to understand the text and generate an appropriate response. Vector embeddings allow the model to capture the semantics and context of the words in the comment, facilitating a more accurate classification of sentiment.
Vector embeddings offer a powerful tool for data analysis and artificial intelligence, with practical applications in a wide range of business areas. Their ability to process and analyze large sets of textual data can provide valuable insights and significantly improve decision-making and operational efficiency in companies.
Be the first to comment