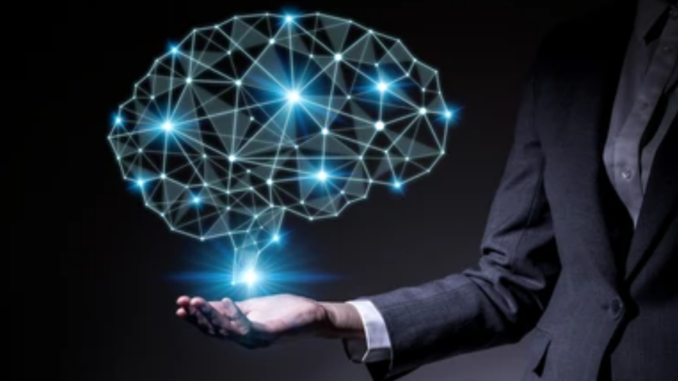
Neural networks form the backbone of modern machine learning, enabling the creation of powerful models that can learn complex patterns from data. In TensorFlow, one of the most popular deep learning frameworks, there are several approaches to building neural network models. In this article, we’ll explore four primary methods: Sequential Model, Functional API, Model Subclassing, and Custom Layers.
A sequential model is a linear stack of layers used for building simple neural network architectures. It represents a straightforward way to create a neural network where each layer is added sequentially and the data flows sequentially through each layer. This type of model is suitable for feedforward neural networks where the information passes through the layers in a fixed order, from the input layer through hidden layers to the output layer.
Example:
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense# Define a Sequential model
model = Sequential()
# Add layers to the model
model.add(Dense(units=64, activation='relu', input_shape=(input_dim))
model.add(Dense(units=10, activation='softmax'))
In this example:
- The
Sequential()
function initializes an empty sequential model. - The
add()
method is used to add layers to the model one by one. - The first layer added (
Dense
) specifies the number of units (neurons), activation function (‘relu’ in this case), and input shape. - The second layer is the output layer with 10 units and a ‘softmax’ activation function, which is commonly used for multiclass classification problems.
The Sequential model is convenient for building simple models quickly, but it may not be suitable for more complex architectures with multiple inputs, shared layers, or non-sequential connections. In such cases, the Functional API or Model Subclassing can be more appropriate.
The Functional API in TensorFlow is an interface for building complex and flexible neural network architectures. Unlike the Sequential API, which is linear and straightforward, the Functional API allows for more intricate models with multiple inputs, multiple outputs, shared layers, and non-sequential connections. It is a powerful and versatile way to create neural networks and is often used for more advanced tasks in deep learning.
from tensorflow.keras.layers import Input, Dense
from tensorflow.keras.models import Model# Define input layer
input_layer = Input(shape=(input_dim,))
# Create hidden layer
hidden_layer = Dense(units=64, activation='relu')(input_layer)
# Create output layer
output_layer = Dense(units=10, activation='softmax')(hidden_layer)
# Define the model
model = Model(inputs=input_layer, outputs=output_layer)
# Compile the model and specify loss, optimizer, etc.
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
# Print model summary
model.summary()
The Functional API in TensorFlow is an interface for building complex and flexible neural network architectures. Unlike the Sequential API, which is linear and straightforward, the Functional API allows for more intricate models with multiple inputs, multiple outputs, shared layers, and non-sequential connections. It is a powerful and versatile way to create neural networks and is often used for more advanced tasks in deep learning.
Key features and concepts of the Functional API include:
- Explicit Input and Output Handling:
- In the Functional API, you explicitly define input layers and output layers, creating a directed acyclic graph of layers.
- This allows for models with multiple input tensors and multiple output tensors.
- Layer Connection:
- Layers are connected by calling a layer on a tensor, just like a function. The output tensor of one layer becomes the input tensor for the next.
- This connection is explicitly defined, providing greater flexibility in designing complex neural network architectures.
Shared Layers:
- The Functional API supports the creation of models with shared layers, where the same layer is applied to multiple inputs or is reused in different parts of the model.
Here’s a simple example demonstrating the Functional API:
from tensorflow.keras.layers import Input, Dense
from tensorflow.keras.models import Model
# Create hidden layer
hidden_layer = Dense(units=64, activation='relu')(input_layer)
# Create output layer
output_layer = Dense(units=10, activation='softmax')(hidden_layer)
# Define the model
model = Model(inputs=input_layer, outputs=output_layer)
# Compile the model and specify loss, optimizer, etc.
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
# Print model summary
model.summary()
In this example
Input
, Dense
, and other layers are explicitly connected to create a model using the Functional API. The resulting model is more flexible and can handle a variety of architectures, making it suitable for complex tasks in deep learning.
Model Subclassing in TensorFlow is a way to create custom neural network models by subclassing the tf.keras.Model
class. This approach provides the highest level of flexibility in defining custom architectures and behaviors for your neural networks. Unlike the Sequential API or the Functional API, Model Subclassing allows you to define the forward pass of the model explicitly by overriding the call
method.
from tensorflow.keras import layers, Modelclass MyModel(Model):
def __init__(self):
super(MyModel, self).__init__()
self.dense1 = layers.Dense(64, activation='relu')
self.dense2 = layers.Dense(10, activation='softmax')
def call(self, inputs):
x = self.dense1(inputs)
return self.dense2(x)
Model Subclassing is particularly useful when building highly customized models, introducing dynamic behavior, or implementing complex architectures that cannot be easily expressed with the Sequential or Functional API. However, it requires careful management of the forward pass and may involve more manual coding compared to other APIs.
Custom layers in TensorFlow allow you to define your own layer with custom functionality that is not covered by existing layers in the framework. Creating custom layers provides a way to implement unique operations, non-standard activations, or any specialized functionality you may require for your neural network model. To create a custom layer, you typically subclass the tf.keras.layers.Layer
class and implement the build
and call
methods.
import tensorflow as tfclass MyCustomLayer(tf.keras.layers.Layer):
def __init__(self, output_dim, **kwargs):
self.output_dim = output_dim
super(MyCustomLayer, self).__init__(**kwargs)
def build(self, input_shape):
self.kernel = self.add_weight("kernel", (input_shape[1], self.output_dim))
super(MyCustomLayer, self).build(input_shape)
Choosing the right approach for building neural network models in TensorFlow depends on the complexity of the task at hand. The Sequential Model is excellent for simple, linear architectures, while the Functional API provides flexibility for more complex models. Model Subclassing and Custom Layers offer unparalleled customization for advanced scenarios. Understanding these approaches empowers developers to select the best fit for their specific requirements, striking a balance between simplicity and flexibility in model design.
Be the first to comment