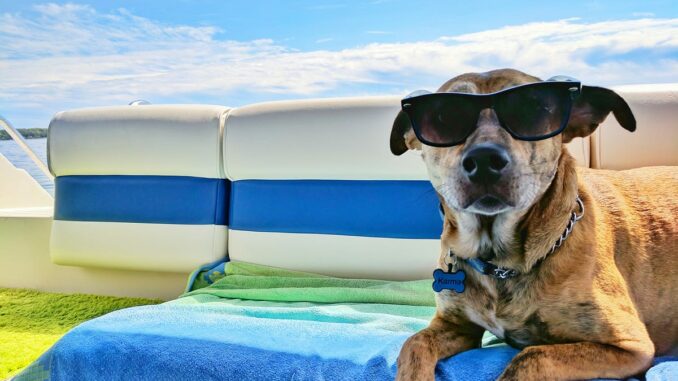
Here are the overall steps involved in building our first model:
- Load the Dataset.
2. Define Model.
3. Compile Model.
4. Fit Model.
5. Evaluate Model.
The dataset used for building our model is Pima Indians Diabetes Database
So, before building our model let us first read and understand the dataset.
It describes patient medical record data for Pima Indians and whether they had an onset of diabetes within five years. It is a binary classification problem (onset of diabetes as 1 or not as 0). The input variables that describe each patient are numerical and have varying scales.
Attributes in the dataset are:
- Number of times pregnant.
- Plasma glucose concentration.
3. Diastolic blood pressure (mm Hg).
4. Triceps skin fold thickness (mm).
5. 2-Hour serum insulin (mu U/ml).
6. Body mass index.
7. Diabetes pedigree function.
8. Age (years).
9. Class, onset of diabetes within five years
All attributes are numerical makes it easy to use directly with neural networks that expect numerical inputs and output values, and ideal for our first neural network in Keras.
Whenever we build a model that uses a stochastic process (e.g. random numbers), it is suggested to initialize the random number generator with a fixed seed value.
Why to use fixed seed value?
Basically, by setting up the fixed seed value the code tends to give the same result again and again if we run the code again and again.
So, this will be useful if you need to demonstrate a result, compare algorithms using the same source of randomness, or debug a part of your code.
kindly skip the below one-liner, if you already have keras installed in your environment. If not, try the below code:
!pip install keras
Here you go!!
from keras.models import Sequential
from keras.layers import Dense
import numpy# fix random seed for reproducibility
seed = 7
numpy.random.seed(seed)
Before loading the dataset first we need to season the dataset.
After unzipping the folder you need to remove the line containing the column names, or else you’ll face errors.
Now, you can load the dataset using numpy:
# load pima indians dataset
dataset = numpy.loadtxt("pima-indians-diabetes.csv", delimiter=",")
# split into input (X) and output (Y) variables
X = dataset[:,0:8]
Y = dataset[:,8]
Once loaded we can split the dataset into input variables (X) and the output class variable (Y).
Models in Keras are defined as a sequence of layers. We create a Sequential model and add layers one at a time until we are happy with our network topology. The first thing to get right is to ensure the input layer has the right number of inputs. This can be specified when creating the first layer with the input dim argument and setting it to 8 for the 8 input variables.
How do we know the number of layers to use and their types? This is a very hard question. There are heuristics that we can use and often the best network structure is found through a process of trial and error experimentation.
In this example, we will use a fully connected network structure with three layers. Fully connected layers are defined using the Dense class. We can specify the number of neurons in the layer as the first argument, and specify the activation function using the activation argument.
We will use the rectifier (relu) activation function on the first two layers and the sigmoid activation function on the output layer.
We use a sigmoid activation function on the output layer to ensure our network output is between 0 and 1 and easy to map to either a probability of class 1 or snap to a hard classification of either class with a default threshold of 0.5.
The first hidden layer has 12 neurons and expects 8 input variables. The second hidden layer has 8 neurons and finally, the output layer has 1 neuron to predict the class (onset of diabetes or not).
#Creating a model
model = Sequential()
model.add(Dense(12,activation="relu",input_dim=8))
model.add(Dense(8,activation="relu"))
model.add(Dense(1,activation="sigmoid"))
Compiling the model uses efficient numerical libraries under the covers (the so-called backend) such as Theano or TensorFlow. The backend automatically chooses the best way to represent the network for training and making predictions to run on your hardware.
When compiling, we must specify some additional properties required when training the network. Remember training a network means finding the best set of weights to make predictions for this problem.
We must specify the loss function to use to evaluate a set of weights, the optimizer used to search through different weights for the network, and any optional metrics we would like to collect and report during training.
In this case, we will use BinaryCrossentropy
,cross-entropy loss between true labels and predicted labels. We will also use the efficient gradient descent algorithm ‘adam’ for no other reason that it is an efficient default.
# Compile model
model.compile(loss='BinaryCrossentropy', optimizer='adam', metrics=['accuracy'])
We can train or fit our model on our loaded data by calling the fit() function on the model.
The training process will run for a fixed number of iterations through the dataset called epochs, which we must specify using the epochs argument. We can also set the number of instances that are evaluated before a weight update in the network is performed called the batch size and set using the batch size argument.
# Fit the model
model.fit(X, Y, nb_epoch=150, batch_size=10)
It’s time to evaluate our model.
This will only give us an idea of how well we have modeled the dataset (e.g. train accuracy), but no idea of how well the algorithm might perform on new data.
But ideally, you could separate your data into train and test datasets for the training and evaluation of your model.
You can evaluate your model on your training dataset using the evaluation() function on your model and pass it to the same input and output used to train the model. This will generate a prediction for each input and output pair and collect scores, including the average loss and any metrics you have configured, such as accuracy.
# evaluate the model
scores = model.evaluate(X, Y)
print("%s: %.2f%%" % (model.metrics_names[1], scores[1]*100))
Magic is over— Our first Neural Network is built!!
Hope you like this page…….
Be the first to comment